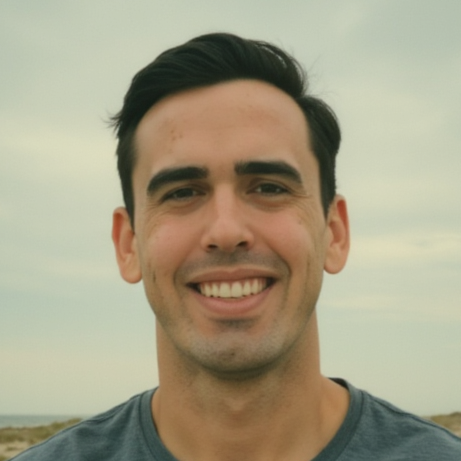
Game Developer Interview Preparation
Interview Preparation Guide for Game Developer Position
Overview of Required and Recommended Certifications, Educational Background, and Industry Qualifications
To enhance a candidate’s profile for a Game Developer position, consider the following educational backgrounds, certifications, and qualifications:
-
Educational Background:
- Bachelor’s Degree in Computer Science or Game Development: A solid foundation in programming, algorithms, and software engineering practices.
- Master’s Degree: Specializations in computer graphics, AI, or interactive media can be advantageous.
-
Certifications:
- Unity Certified Developer: Validates your expertise in the Unity engine, which is widely used in game development.
- Unreal Engine Certification: Demonstrates proficiency in the Unreal Engine, another popular game development platform.
- Programming Certifications: Such as C++ Certified Professional Programmer for proficiency in C++.
-
Industry Qualifications:
- Portfolio of Projects: Demonstrating experience with game development projects, either independently or as part of a team.
- Participation in Game Jams: Shows ability to work under pressure and deliver creative solutions quickly.
- Contributions to Open Source Projects: Demonstrates collaboration skills and a commitment to the community.
Interview Questions and Answers
1. What is the importance of object-oriented programming (OOP) in game development?
Answer:
-
Context: Object-oriented programming is a paradigm centered around objects rather than actions, and data rather than logic.
-
Importance in Game Development:
- Encapsulation: Allows for modular code, where game entities (e.g., characters, items) have defined interfaces, making the system more manageable.
- Inheritance: Facilitates the reuse of code. For example, a generic ‘Enemy’ class can be extended to specific types like ‘Zombie’ or ‘Alien’.
- Polymorphism: Enables objects to be treated as instances of their parent class, allowing for flexible and reusable code. For example, different enemy types can be managed through a single interface.
-
Real-World Scenario:
- In a game where you have multiple types of characters, using OOP allows for creating a base class
Character
with common properties like health and position. Different characters likePlayer
andNPC
can inherit fromCharacter
, adding their specific behaviors.
- In a game where you have multiple types of characters, using OOP allows for creating a base class
-
Best Practices:
- Use inheritance judiciously to avoid deep and complex hierarchies.
- Prefer composition over inheritance when appropriate, to achieve more flexibility.
-
Common Pitfalls:
- Overusing inheritance can lead to rigid structures that are hard to refactor.
- Avoid tight coupling; changes in one class should not ripple across the system.
-
Follow-Up Points:
- Discuss the use of design patterns such as the Component pattern, often used in game engines like Unity.
2. Can you explain how you would optimize a game for performance?
Answer:
-
Optimization Techniques:
- Profiling: Identify bottlenecks using profiling tools to focus optimization efforts. For example, Unity’s Profiler or Unreal’s Stats system.
- Level of Detail (LOD): Implement LOD for 3D models to reduce polygon count based on the camera distance.
- Efficient Algorithms: Use data structures and algorithms that minimize computational overhead. For example, choosing the right pathfinding algorithm (e.g., A* over Dijkstra for grid-based maps).
- Memory Management: Optimize memory usage by pooling objects and managing resources efficiently.
-
Real-World Scenario:
- In a large open-world game, utilize LOD systems to decrease the rendering load by displaying detailed models only when necessary. Implement culling techniques to avoid rendering objects outside the player’s view.
-
Common Pitfalls:
- Premature optimization can lead to wasted effort and more complex code. Focus on profiling first.
- Over-optimization might compromise code readability and maintainability.
-
Follow-Up Points:
- Discuss specific experiences with optimizing games for different platforms, such as mobile vs. console.
3. Describe a challenging bug you encountered in a game project and how you resolved it.
Answer:
-
Context: Bugs in game development can range from minor graphical glitches to critical gameplay issues.
-
Example:
- Bug Description: A physics bug where characters would occasionally clip through walls.
- Resolution Process:
- Reproduce the Bug: Isolated the conditions under which the bug occurred.
- Diagnose: Used debugging tools to track character collision detection and response.
- Solution: Identified that the collision detection system was missing edge cases. Enhanced the collision detection algorithm to account for high-speed movements and adjusted the physics step to prevent tunneling.
- Testing: Conducted extensive testing across different levels to ensure the fix was robust.
-
Alternative Considerations:
- Implement continuous collision detection for fast-moving objects.
- Use physics middleware with better out-of-the-box solutions for complex simulations.
-
Common Pitfalls:
- Fixing symptoms rather than root causes can lead to recurring issues.
- Inadequate testing after the fix can result in regressions.
-
Follow-Up Points:
- Discuss collaborative efforts with QA teams and other developers to ensure comprehensive testing and validation.
4. How do you approach designing a game level?
Answer:
-
Design Process:
- Conceptualization: Start with a clear vision and objectives for the level. Define the theme and mood.
- Layout Design: Sketch a rough layout focusing on player flow and pacing. Determine key areas and points of interest.
- Iteration and Prototyping: Build a basic prototype to test gameplay mechanics and flow. Use feedback to iterate.
- Detailing and Polish: Add environmental details, textures, lighting, and sound to enhance immersion.
-
Real-World Scenario:
- In a stealth-based game, design levels with multiple paths and cover opportunities. Balance the level to accommodate different playstyles, such as aggressive or stealthy approaches.
-
Best Practices:
- Ensure levels have a clear narrative and progression.
- Balance challenge and accessibility to cater to a wide range of players.
-
Common Pitfalls:
- Overcomplicating level design can overwhelm players and disrupt flow.
- Neglecting playtesting can lead to unbalanced or frustrating player experiences.
-
Follow-Up Points:
- Discuss experiences with player feedback and how it influenced level design decisions.
5. What strategies do you use to keep up with the latest trends and technologies in game development?
Answer:
-
Strategies:
- Continuous Learning: Attend industry conferences, workshops, and webinars. Engage with platforms like GDC (Game Developers Conference).
- Online Courses and Tutorials: Platforms like Coursera, Udemy, and YouTube offer tutorials on emerging technologies and techniques.
- Networking: Join online communities and forums, such as Reddit’s game development section or Unity/Unreal community forums.
- Experimentation: Develop small projects or prototypes to explore new tools and techniques.
-
Real-World Scenario:
- Upon learning about ray tracing technology, undertake a personal project to implement it in a small-scale game, gaining practical experience with its benefits and challenges.
-
Common Pitfalls:
- Overwhelming oneself with too many new technologies can dilute focus and mastery.
- Neglecting to apply new knowledge practically can result in superficial understanding.
-
Follow-Up Points:
- Discuss specific technologies or trends that have recently caught your attention and how they could be applied to future projects.
6. Explain the role of a game engine in development and why you might choose one engine over another.
Answer:
-
Role of a Game Engine:
- Framework and Tools: Provides a suite of tools for rendering graphics, handling physics, scripting, and more.
- Efficiency: Streamlines development by offering pre-built functionalities and reducing the need for building systems from scratch.
- Cross-Platform Support: Many engines offer support for multiple platforms, easing the porting process.
-
Choosing an Engine:
- Unity: Known for versatility and a strong asset store. Ideal for 2D and 3D games, with a large community and extensive documentation.
- Unreal Engine: Offers high-quality graphics and is often used for AAA titles. Known for the Blueprint visual scripting system.
- Godot: Open-source and lightweight, suitable for indie developers. Offers a straightforward scripting language (GDScript).
-
Real-World Scenario:
- For a mobile game with simple graphics and a need for rapid prototyping, Unity might be preferred for its ease of use and strong 2D support.
- For a high-fidelity VR experience, Unreal Engine may be chosen for its superior rendering capabilities.
-
Common Pitfalls:
- Over-relying on an engine’s default settings can lead to performance issues if not optimized for specific needs.
- Not fully understanding an engine’s capabilities can lead to underutilization of its features.
-
Follow-Up Points:
- Discuss any custom modifications or plugins you have developed or used to extend an engine’s capabilities.
7. How do you handle player feedback during the game development process?
Answer:
-
Handling Feedback:
- Collection: Use surveys, forums, and playtesting sessions to gather feedback from a diverse player base.
- Analysis: Categorize feedback into actionable insights and prioritize based on impact and feasibility.
- Implementation: Make iterative changes, focusing on critical feedback that aligns with the game’s vision.
- Communication: Keep an open line of communication with the community about what feedback has been considered and implemented.
-
Real-World Scenario:
- During a beta test, players report that a boss fight is too difficult. Analyze difficulty metrics and player progression data to adjust the boss’s mechanics, ensuring it remains challenging but fair.
-
Best Practices:
- Balance player feedback with the game’s design goals. Not all feedback will align with the intended experience.
- Engage with the community to build trust and transparency.
-
Common Pitfalls:
- Ignoring feedback or being overly defensive can alienate your player base.
- Implementing all feedback without discernment can dilute the game’s core vision.
-
Follow-Up Points:
- Discuss specific instances where player feedback significantly improved a project and how it was handled.
8. Describe a time when you had to work under a tight deadline. How did you manage it?
Answer:
-
Context: Tight deadlines are common in game development, especially nearing launch dates or major updates.
-
Example:
- Situation: Asked to deliver a critical game patch in one week due to a game-breaking bug discovered post-launch.
- Action: Prioritized tasks by urgency and impact. Collaborated closely with team members to divide workload effectively.
- Tools Used: Utilized project management tools like JIRA to track progress and communicate updates.
- Outcome: Successfully delivered the patch on time, restoring game functionality and maintaining player satisfaction.
-
Alternative Considerations:
- Automated testing could have been increased to prevent similar issues.
- Considered delaying non-critical features to focus resources effectively.
-
Common Pitfalls:
- Compromising quality for speed can lead to further issues down the line.
- Poor communication can result in duplicated efforts or overlooked tasks.
-
Follow-Up Points:
- Discuss stress management techniques and how you ensure quality under pressure.
9. How do you ensure code quality and maintainability in your projects?
Answer:
-
Ensuring Code Quality:
- Code Reviews: Regular peer reviews to catch potential issues early and share knowledge.
- Consistent Style: Adhere to coding standards and guidelines to maintain consistency across the codebase.
- Automated Testing: Implement unit tests and integration tests to catch regressions and ensure functionality.
- Documentation: Maintain thorough documentation for code, APIs, and systems to aid future development and onboarding.
-
Real-World Scenario:
- On a project with frequent team changes, established a set of coding guidelines and automated linting tools to ensure new members could quickly contribute effectively.
-
Best Practices:
- Refactor code periodically to address technical debt and improve readability.
- Invest time in creating a robust testing pipeline to automate repetitive checks.
-
Common Pitfalls:
- Neglecting code reviews can lead to inconsistent and error-prone code.
- Over-documenting can be as problematic as under-documenting, leading to outdated or ignored documentation.
-
Follow-Up Points:
- Discuss tools and practices used for continuous integration and deployment in game development.
10. What are the key considerations when developing a multiplayer game?
Answer:
-
Key Considerations:
- Networking Architecture: Choose between client-server and peer-to-peer models based on game requirements. Client-server is more secure and reliable for most games.
- Latency and Synchronization: Implement techniques like client-side prediction and server reconciliation to manage latency and ensure smooth gameplay.
- Scalability: Design systems to handle a large number of concurrent players, with load balancing and efficient resource management.
- Cheat Prevention: Secure communication and validate actions server-side to prevent cheating.
-
Real-World Scenario:
- For an online shooter, opted for a client-server model to ensure centralized control over game state, reducing the risk of cheating and improving reliability.
-
Best Practices:
- Simulate high-latency environments during testing to refine synchronization techniques.
- Regularly update security protocols to protect against emerging threats.
-
Common Pitfalls:
- Ignoring network bandwidth limitations can lead to poor performance and player frustration.
- Overlooking security can lead to exploits and a compromised player experience.
-
Follow-Up Points:
- Discuss specific technologies or frameworks used for networking in multiplayer games.
11. Can you explain the difference between real-time and turn-based game systems? Provide examples.
Answer:
-
Real-Time Systems:
- Definition: Actions occur continuously and simultaneously, requiring players to make quick decisions.
- Examples:
- StarCraft: Players manage resources and units in real-time to outmaneuver opponents.
- Overwatch: A first-person shooter where gameplay requires fast reflexes and real-time strategy.
- Considerations: Requires careful balance to ensure fairness despite different player skill levels.
-
Turn-Based Systems:
- Definition: Players take turns making moves, allowing for strategic planning without time pressure.
- Examples:
- Civilization: Players take turns to manage their empire, focusing on long-term strategy.
- XCOM: Turn-based tactics game where players plan each move carefully.
- Considerations: Balancing player turns to prevent downtime and maintaining engagement is crucial.
-
Real-World Scenario:
- In developing a hybrid game, real-time elements were used for exploration, while turn-based systems were used for combat to blend strategy with action.
-
Best Practices:
- Real-time games need efficient input handling and responsive controls.
- Turn-based games should focus on providing meaningful choices and strategic depth.
-
Common Pitfalls:
- Real-time games can suffer from performance issues if not optimized for fast-paced action.
- Turn-based games risk becoming tedious if turns take too long or offer limited strategic options.
-
Follow-Up Points:
- Discuss the challenges and solutions when mixing real-time and turn-based elements in a single game.
12. How do you approach balancing gameplay mechanics?
Answer:
-
Balancing Approach:
- Data Collection: Use analytics to gather data on player behavior and outcomes.
- Iterative Testing: Continuously test and adjust mechanics based on player feedback and data insights.
- Playtesting: Conduct playtesting sessions with diverse player groups for varied perspectives.
- Metrics and Tuning: Define key metrics (e.g., win/loss ratios, player progression speed) to guide balancing efforts.
-
Real-World Scenario:
- In a MOBA game, character abilities and stats were continuously monitored and adjusted based on player performance data to ensure no character became overpowered.
-
Best Practices:
- Use a combination of quantitative data and qualitative feedback to inform balancing decisions.
- Early and frequent testing is crucial to catch imbalances before they affect the player experience.
-
Common Pitfalls:
- Ignoring player feedback can result in imbalances that detract from the game experience.
- Relying solely on data without context can lead to misinterpretations.
-
Follow-Up Points:
- Discuss specific tools or methods used for tracking and analyzing gameplay data.
13. What is the significance of narrative in game design, and how do you integrate it effectively?
Answer:
-
Significance of Narrative:
- Engagement: A well-crafted narrative can immerse players and enhance emotional investment.
- Context: Provides context for player actions and in-game mechanics, making them more meaningful.
- Motivation: Drives progression and motivates players to complete objectives.
-
Integration Strategies:
- Story-Driven Design: Develop gameplay mechanics that complement and enhance the story.
- Environmental Storytelling: Use the game world itself to convey narrative elements without intrusive exposition.
- Character Development: Create compelling characters with clear motivations and arcs that resonate with players.
-
Real-World Scenario:
- In an RPG, narrative was integrated through branching dialogue systems and player choices that impacted the story, creating a personalized experience.
-
Best Practices:
- Balance narrative with gameplay to ensure neither detracts from the other.
- Use pacing effectively to maintain interest and avoid narrative fatigue.
-
Common Pitfalls:
- Overloading the player with information can lead to disengagement.
- Inconsistent narrative elements can break immersion.
-
Follow-Up Points:
- Discuss experiences with narrative design and collaboration with writers and narrative designers.
14. How do you ensure accessibility in your game designs?
Answer:
-
Ensuring Accessibility:
- Inclusive Design: Consider diverse player needs from the start, including visual, auditory, and motor impairments.
- Customization Options: Provide adjustable settings for controls, difficulty, subtitles, and colorblind modes.
- Feedback and Testing: Engage with players with disabilities to gather feedback and test accessibility features.
-
Real-World Scenario:
- Implemented a customizable control scheme in a platformer game, allowing players to remap controls and adjust sensitivity, accommodating various physical abilities.
-
Best Practices:
- Follow established accessibility guidelines, such as those provided by the International Game Developers Association (IGDA).
- Prioritize accessibility during the early stages of development to avoid costly retrofits.
-
Common Pitfalls:
- Treating accessibility as an afterthought can lead to incomplete or ineffective solutions.
- Assuming all players have the same needs without conducting proper research and testing.
-
Follow-Up Points:
- Discuss specific accessibility features implemented in past projects and their impact on player inclusivity.
15. Explain the use of artificial intelligence (AI) in game development.
Answer:
-
AI in Game Development:
- NPC Behavior: AI is used to control non-player character (NPC) behavior, making them appear intelligent and responsive.
- Pathfinding: Implement algorithms like A* for efficient pathfinding in complex environments.
- Procedural Generation: Use AI to generate content, such as levels or quests, dynamically.
-
Real-World Scenario:
- Developed an AI system for enemy NPCs in a stealth game, enabling them to patrol, investigate noises, and coordinate with each other to provide a challenging experience.
-
Best Practices:
- Balance AI difficulty to ensure it is challenging but fair, avoiding frustration.
- Optimize AI systems to prevent performance issues, especially in large-scale environments.
-
Common Pitfalls:
- Overly predictable AI can lead to repetitive and unengaging gameplay.
- Complex AI systems can become resource-intensive, impacting performance.
-
Follow-Up Points:
- Discuss experiences with AI middleware and custom AI solutions in game projects.
16. How do you address cultural sensitivity in game design?
Answer:
-
Addressing Cultural Sensitivity:
- Research and Consultation: Conduct thorough research and consult with cultural experts to ensure accurate representation.
- Diverse Perspectives: Involve a diverse team in the design process to provide multiple viewpoints and reduce biases.
- Respectful Representation: Avoid stereotypes and ensure cultures are represented authentically and respectfully.
-
Real-World Scenario:
- In a historical game set in multiple cultures, worked with cultural consultants to ensure clothing, language, and customs were depicted accurately, enhancing authenticity and respect.
-
Best Practices:
- Continuously educate the team on cultural issues and encourage open dialogue.
- Test content with diverse audiences to identify potential sensitivities.
-
Common Pitfalls:
- Relying on stereotypes or clichés can lead to cultural insensitivity.
- Neglecting to address cultural issues can alienate players and damage the game’s reputation.
-
Follow-Up Points:
- Discuss experiences with cultural consultants and the impact on game design.
17. What are the challenges and solutions in cross-platform game development?
Answer:
-
Challenges:
- Technical Differences: Varying hardware capabilities and input methods across platforms.
- Performance Optimization: Ensuring consistent performance on different platforms.
- Platform-Specific Features: Adapting to unique features or restrictions of each platform.
-
Solutions:
- Cross-Platform Engines: Use engines like Unity or Unreal that support multiple platforms natively.
- Conditional Compilation: Implement platform-specific code using conditional compilation directives.
- Unified Input Systems: Design input systems that can be easily adapted to different devices.
-
Real-World Scenario:
- Developed a game for both console and mobile, using Unity’s cross-platform capabilities to share a common codebase while implementing platform-specific optimizations for performance and controls.
-
Best Practices:
- Test extensively on all target platforms to identify and address specific issues.
- Prioritize feature parity while considering platform strengths and limitations.
-
Common Pitfalls:
- Ignoring platform-specific guidelines can lead to rejection or poor user experiences.
- Overcomplicating the codebase with platform-specific hacks can hinder maintainability.
-
Follow-Up Points:
- Discuss specific experiences with porting games between platforms and the challenges faced.
18. Describe your approach to managing a game development team.
Answer:
-
Management Approach:
- Clear Communication: Establish open lines of communication and regular check-ins to keep the team aligned.
- Defined Goals and Milestones: Set clear objectives and milestones to guide development and measure progress.
- Fostering Collaboration: Encourage collaboration and knowledge sharing among team members.
- Adaptability: Be flexible and willing to adjust plans based on feedback and changing circumstances.
-
Real-World Scenario:
- Managed a diverse team across multiple time zones, using project management tools like Trello and Slack to coordinate efforts and maintain transparency.
-
Best Practices:
- Empower team members by involving them in decision-making and encouraging ownership of tasks.
- Provide regular feedback and recognition to boost motivation and morale.
-
Common Pitfalls:
- Micromanaging can stifle creativity and autonomy.
- Poor planning and communication can lead to missed deadlines and frustration.
-
Follow-Up Points:
- Discuss experiences with remote team management and the tools used to facilitate collaboration.
19. How do you handle conflicts within a development team?
Answer:
-
Conflict Resolution:
- Identify the Issue: Listen to all parties involved to understand the root cause of the conflict.
- Facilitate Discussion: Encourage open dialogue to address concerns and find common ground.
- Collaborative Solutions: Work towards a solution that satisfies all parties while aligning with project goals.
- Follow-Up: Monitor the situation to ensure the solution is effective and prevent future conflicts.
-
Real-World Scenario:
- Resolved a conflict between designers and developers over feature scope by facilitating a meeting to discuss priorities and compromises, resulting in a mutually agreeable solution.
-
Best Practices:
- Create a culture of respect and open communication to prevent conflicts from escalating.
- Address conflicts promptly to maintain team cohesion and productivity.
-
Common Pitfalls:
- Ignoring conflicts can lead to resentment and decreased team performance.
- Taking sides can undermine trust and objectivity.
-
Follow-Up Points:
- Discuss specific conflict resolution strategies used and their outcomes.
20. What is your approach to testing and quality assurance in game development?
Answer:
-
Testing and QA Approach:
- Comprehensive Testing: Conduct a variety of tests, including unit tests, integration tests, and user acceptance testing.
- Automated Testing: Implement automated testing for repetitive tasks to increase efficiency and coverage.
- Bug Tracking and Management: Use bug tracking tools like JIRA or Bugzilla to document and prioritize issues.
- Player Feedback: Gather feedback from beta testers to identify areas for improvement.
-
Real-World Scenario:
- Established a QA process for a multiplayer game, involving regular playtests and automated stress testing to ensure server stability and performance.
-
Best Practices:
- Integrate testing throughout the development cycle to catch issues early.
- Balance automated and manual testing to ensure comprehensive coverage.
-
Common Pitfalls:
- Skimping on testing can lead to critical issues going undetected until post-launch.
- Over-reliance on automated tests without manual testing can miss user experience issues.
-
Follow-Up Points:
- Discuss experiences with specific testing frameworks and tools used in past projects.
More Software Engineering Interview Guides
Explore more interview guides for Technical positions.
AI Engineer Interview Preparation
The AI Engineer Interview Preparation guide equips job seekers with essential skills and knowledge to excel in AI eng...
Back-End Developer Interview Help
Back-End Developer Interview Help equips job seekers with essential strategies and insights to ace their interviews. ...
Mobile App Developer Interview Help
The Mobile App Developer Interview Help guide equips job seekers with essential strategies to excel in interviews. Le...
Blockchain Developer Interview Questions and Answers
This guide offers a comprehensive collection of Blockchain Developer interview questions and answers, designed to hel...
Software Architect Interview Questions and Answers
Discover essential insights with our Software Architect Interview Questions and Answers guide. Tailored for job seeke...
Recent Blog Articles
Check out recent articles from Tustin Recruiting on all things hiring.
How to Implement Structured JSON-LD for Google Jobs
Learn how to implement structured JSON-LD for Google Jobs to improve your job postings and attract more qualified can...
Common Employee Benefits in Orange County, CA Private Sector
Discover common employee benefits offered by private sector employers in Orange County, CA.
10 High-Paying Sales Jobs You Can Get Without a Degree
Discover 10 high-paying sales jobs you can get without a degree, including entry-level roles and opportunities for ca...
When to Follow Up with a Recruiter
Learn when to follow up with a recruiter after submitting your resume and when to wait for best practices.
Exceptional Software Engineer Jobs in Orange County
Discover top software engineer jobs in Orange County. Unlock salary insights, skills needed, and career tips.
Featured Jobs
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Irvine, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive Equipment Finance
- Anaheim Hills, CA
- Employment Type
- FULL_TIME
- Salary
- $75,000-$95,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 02/09/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Junior Account Executive
- Hayward, CA
- Employment Type
- FULL_TIME
- Salary
- $62,330-$79,329/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Sales Operations Coordinator
- Eugene, OR
- Employment Type
- FULL_TIME
- Salary
- $45,156-$58,201/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Account Executive
- Cypress, TX
- Employment Type
- FULL_TIME
- Salary
- $55,000-$70,000/YEAR
- Team and Date
- Equipment Finance
- Posted: 01/29/2025
-
- Company
- Tustin Recruiting
- Title and Location
- Mobile App Developer
- Lakewood, CA
- Employment Type
- FULL_TIME
- Salary
- $85,013-$118,074/YEAR
- Team and Date
- Software
- Posted: 01/29/2025
Ready to find your next great hire?
Let's discuss your hiring needs. With our deep Orange County network and 20+ years of experience, we'll help you find the perfect candidate.
20+ Years Experience
Deep expertise and a proven track record of successful placements.
Direct-Hire Focus
Specialized in permanent placements that strengthen your team for the long term.
Local Market Knowledge
Unmatched understanding of Orange County's talent landscape and salary expectations.
Premium Job Board
Access top Orange County talent through our curated job board focused on quality over quantity.
Tustin Recruiting is for Everyone
At Tustin Recruiting, we are dedicated to fostering an inclusive environment that values diverse perspectives, ideas, and backgrounds. We strive to ensure equal employment opportunities for all applicants and employees. Our commitment is to prevent discrimination based on any protected characteristic, including race, color, ancestry, national origin, religion, creed, age, disability (mental and physical), sex, gender, sexual orientation, gender identity, gender expression, medical condition, genetic information, family care or medical leave status, marital status, domestic partner status, and military and veteran status.
We uphold all characteristics protected by US federal, state, and local laws, as well as the laws of the country or jurisdiction where you work.